MTE Concepts
A No-Code Guide to understanding the Core Concepts of MTE.
The goal of this guide is to provide context to developers who will be implementing MTE for the first time. This guide only contains pseudo-code to convey ideas; it does not show specific implementation details.
101 - Introduction to MTE
What is MTE?
- MicroToken Exchange (MTE)
- Proprietary and patented cryptographic library
- FIPS 140-3 Certified
- Written in C for speed and performance
- Includes library interfaces for all the most popular languages
- C#, Java, Swift, Objective C, JavaScript, Go, Python
How does MTE work?
The core concept of MTE is using the library to create Encoders and Decoders. These objects have methods that can be called to encode data, decode data, check statuses, and more.
Encoders and Decoders work together to encode data, and it is common to set them up on either end of a network (though MTE does not require internet connectivity to function). An Encoder would encode sensitive data before sending it to a synchronized Decoder waiting to decode the encoded data.

Important: MTE does not transmit data. It simply randomizes and replaces the data with random value streams, allowing the application to send the encoded data.
Creating Encoders and Decoders
Creating an Encoder or Decoder requires calling a create method from the MTE library and providing it with three initialization values.
- Entropy: A random value whose entropy will be used to MTE encode payloads. Entropy is the most critical initialization value when creating a new Encoder or Decoder. Values that are low in entropy will provide poor security. Values with high entropy will provide excellent security.
Important: This value should be kept secret and unstored.
- Nonce: A nonce value is any value that is unlikely to repeat. A high-accuracy time stamp is a good example of a nonce.
- PersonalizationString: Any string uniquely identifying an Encoder or Decoder. A GUID is an acceptable personalization string.
Understanding MTE Pairing
MTE Encoders and Decoders created with the same initialization values are considered "paired." That is, the Decoder is able to recover data successfully that was encoded by its paired Encoder.
Encoders and Decoders created with different initialization values will not work together.
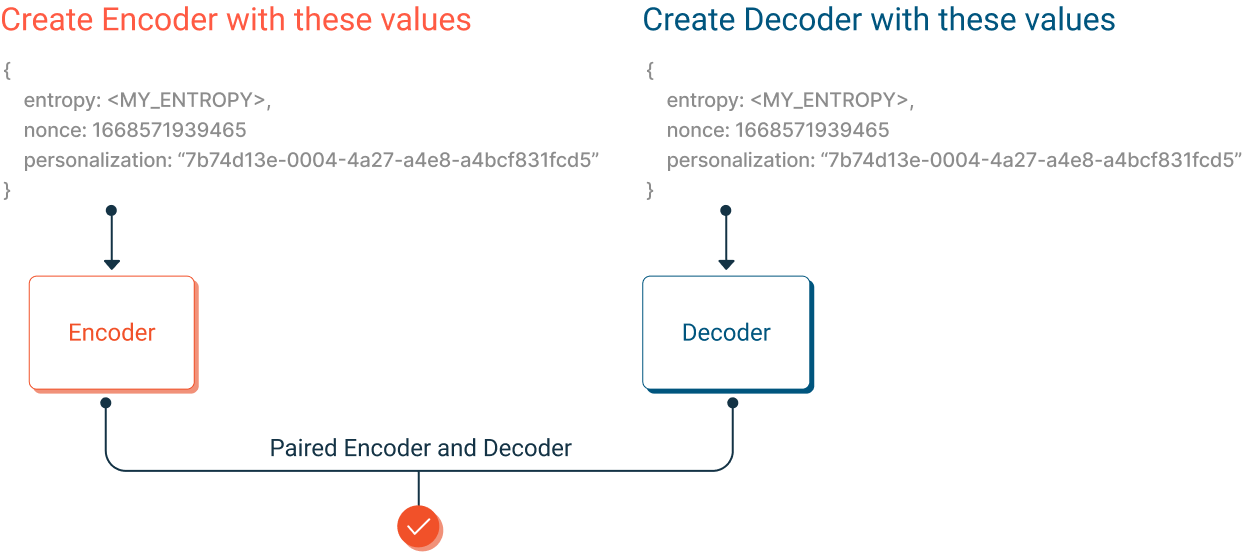
These were created with the same initialization values and are considered "paired." The Decoder can decode data from the Encoder.
Uni-directional Communication
MTE works uni-directionally. Data only flows in one direction, from an Encoder to a Decoder.
To facilitate the request-and-response model of modern client-and-server applications, a developer must set up two pairs of Encoders and Decoders. One Encoder/Decoder pair to send requests, and another Encoder/Decoder pair to send responses.
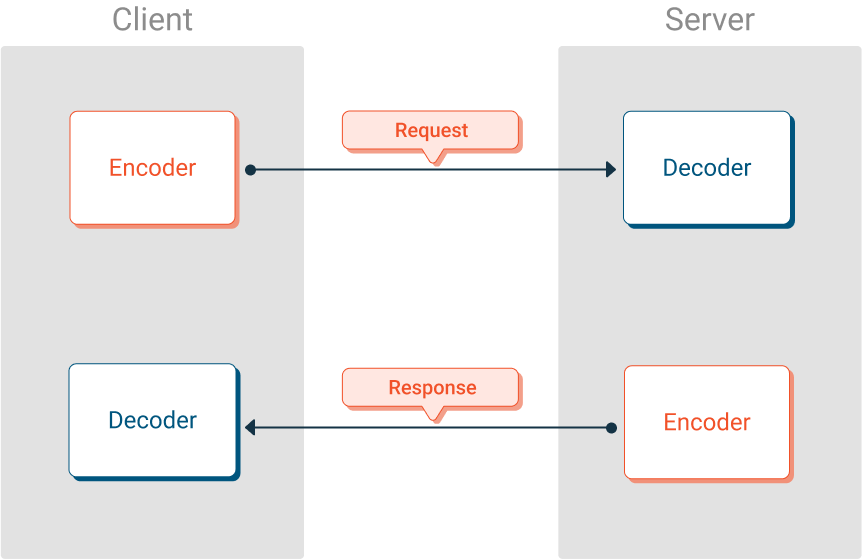
The diagram shows two pairs of Encoders and Decoders working together to allow a client and server application to exchange data securely using MTE.
Important: The red Encoder/Decoder pair used different initialization values than the blue Encoder/Decoder pair. This is an MTE Best Practice that promotes data security.
Create Matching Initialization Values on a Client and Server
Personalization and nonce can be exchanged using a simple network request. We recommend that one side generate the nonce values and the other generate personalization string values.
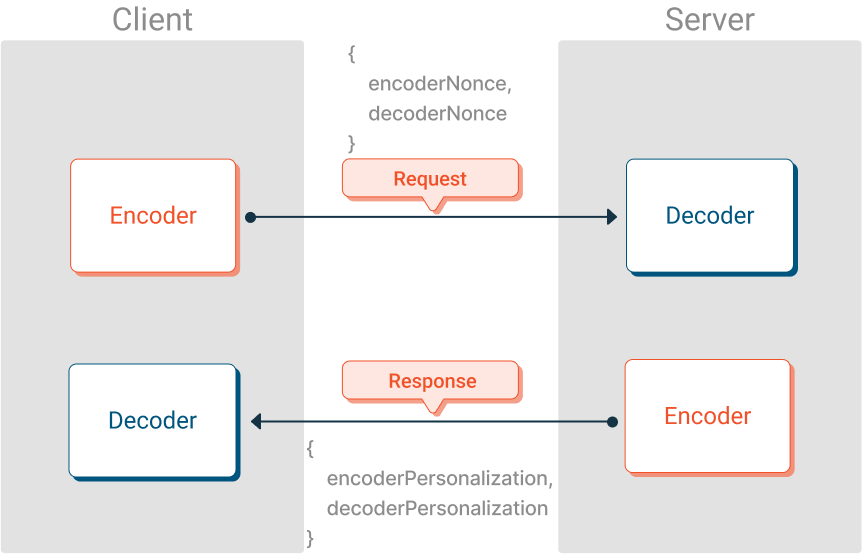
Entropy is too sensitive of a value to send over a network, even with SSL. Instead, the recommended approach is to generate matching values on both the client and the server using Elliptical-Curve Diffie-Helman (ECDH) key exchange.
In ECDH, both the client and server generate public and private keys. They then exchange public keys, and finally, they can combine their keys to generate an identical entropy value on both sides. Public keys can be sent in a normal network request and can even be included in the above request.Eclypses provides an ECDH helper package to ease the effort of this key exchage and ensure consistancy across languages.
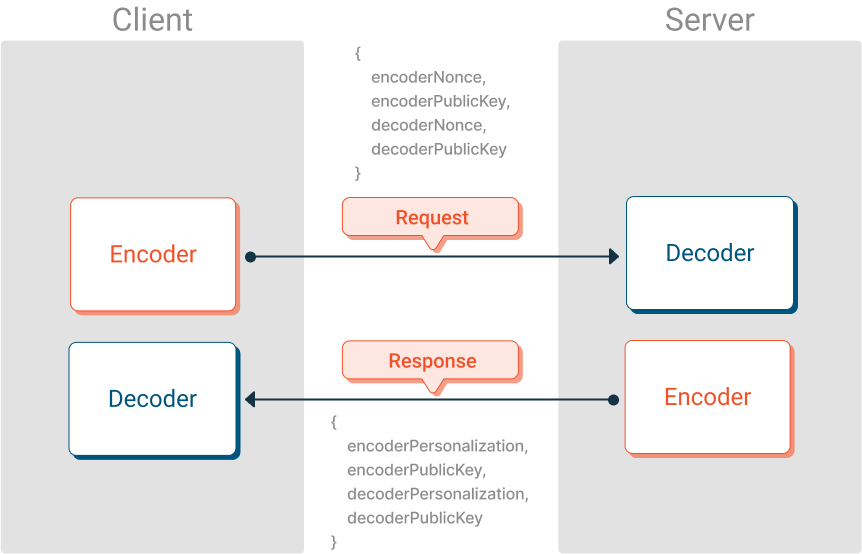
The diagram shows a client app and server app exchanging the information they need to create pairs of Encoders and Decoders. In the diagram, the Encoders and Decoders are not yet created. The values that were exchanged will be used to create them.
Important: The client-Encoder must pair with the server-Decoder (shown in red), and the server-Encoder pairs with the client-Decoder (shown in blue). It is easy to get these mixed up!
102 - MTE State
Initial MTE State
MTE Encoders and Decoders manage their own internal state. When a new Encoder or Decoder is created from its three initialization values, it is said to have its "initial state." The internal state of a paired Encoder and Decoder match, which is what allows them to encode and decode data successfully.
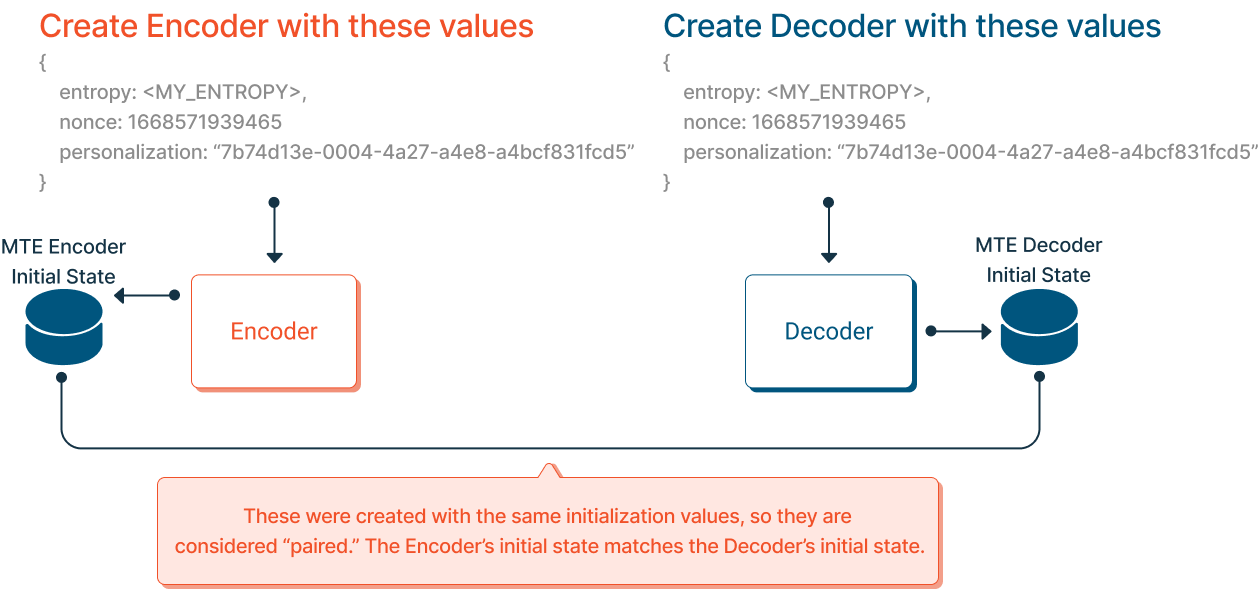
These were created with the same initialization values and are considered "paired." The Encoder's initial state matches the Decoder's initial state.
New State after each Encode / Decode
Each time an Encoder encodes data, its internal state progresses to a new and unique value. Similarly, Decoders' internal states are also progressed after each decode operation.
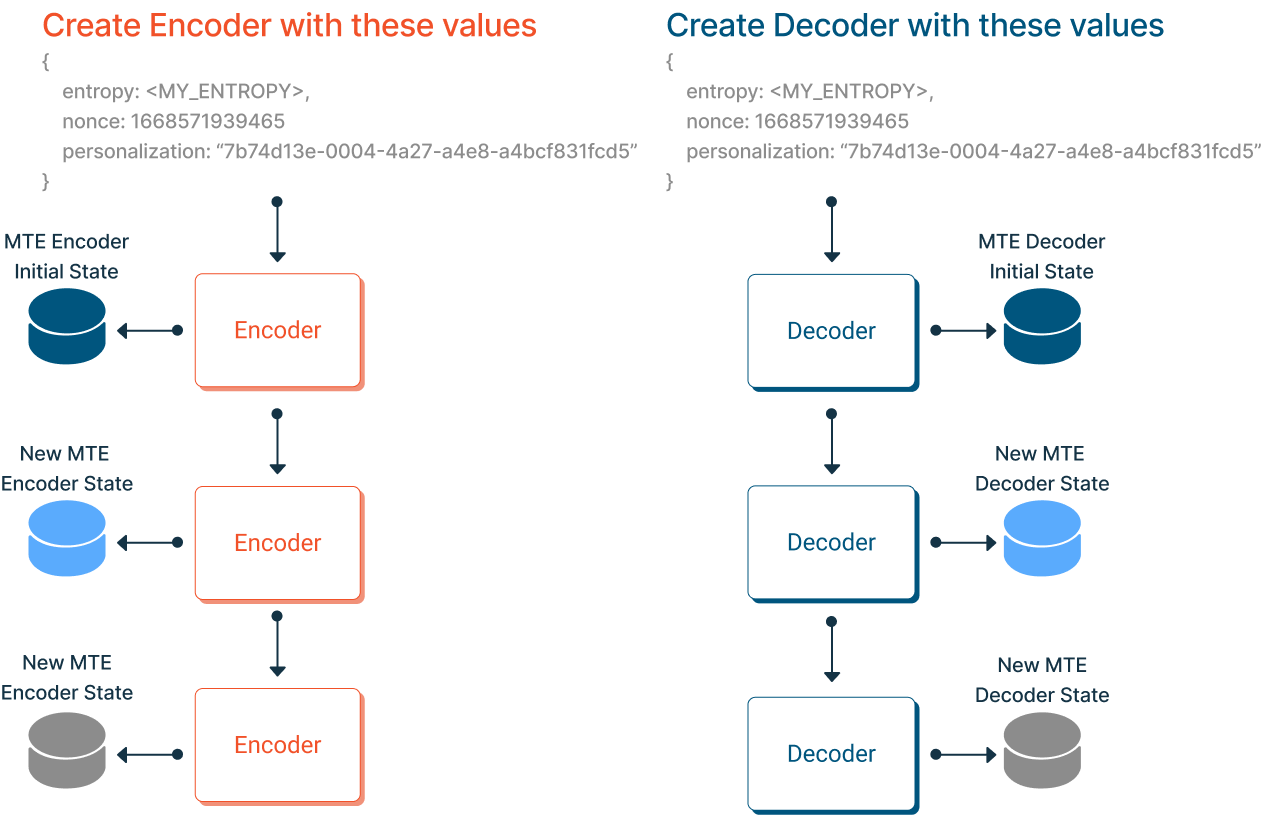
State Synchronization
When a paired Encoder and Decoder are first created, their internal states are synchronized, meaning they have the same internal state. If, at any point, their states get out of sync, the Decoder will no longer be able to decode data from the Encoder. Their states must remain synchronized!
MTE provides methods to help keep Encoder and Decoder States in sync, and MTE also provides methods to deal with out-of-order packet transmissions. We will see these methods in future lessons.
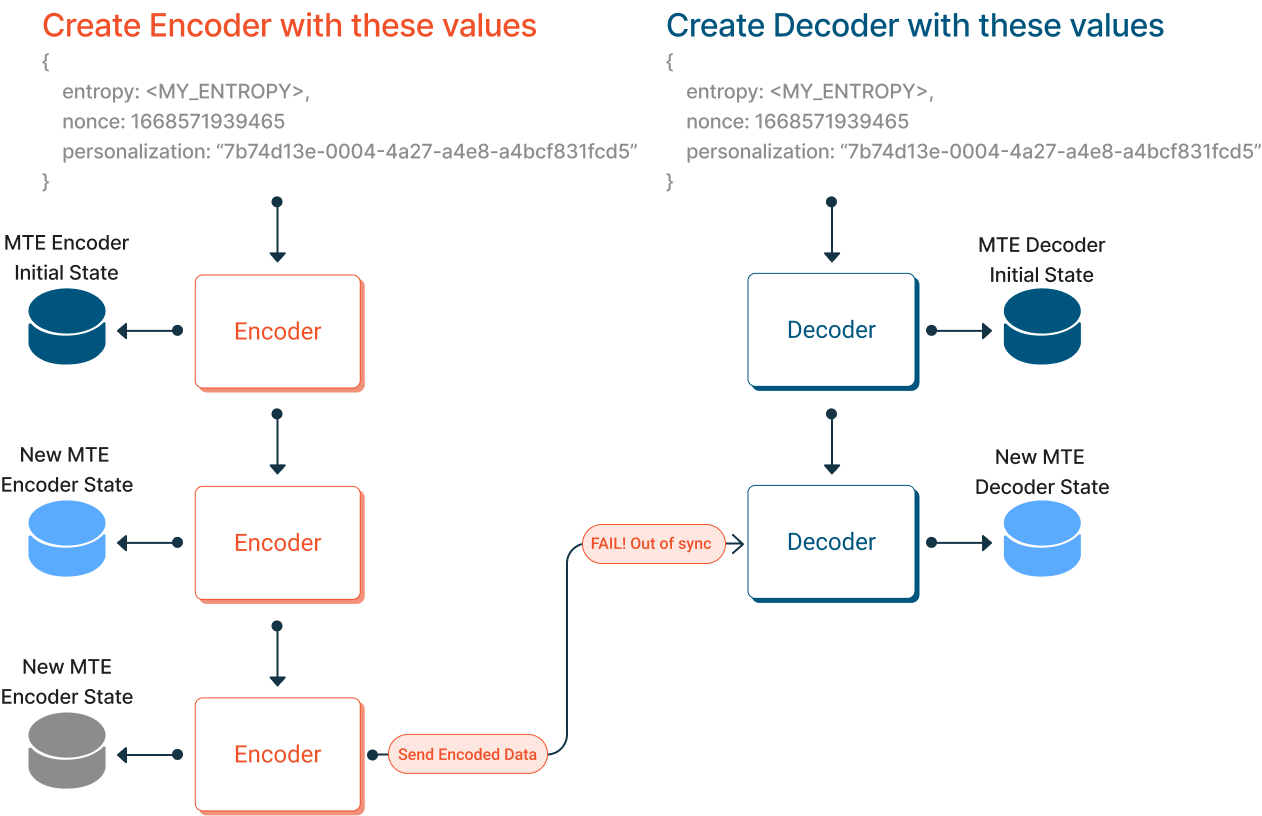
In the above scenario, the Encoder sends its third encoded payload to its paired Decoder, which is only on its second decryption event. This decode operation will fail because the Encoder's and Decoder's internal states are out of sync. This could happen due to an unintentionally dropped network packet that the Decoder never received.
Saving MTE State
Encoders and Decoders can export their internal state, which can then be saved into persistent storage. The state can be exported and saved as a Base64 encoded string or binary.
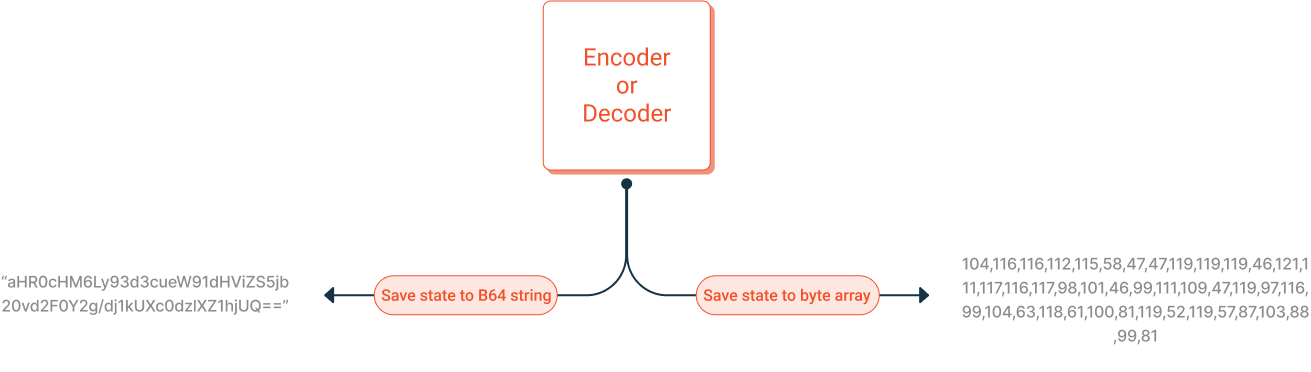
IMPORTANT: MTE State is sensitive data that should be treated like a secret.
Restore Encoder/Decoder from Saved State
An MTE Encoder or Decoder can be restored from a previously saved state, allowing it to resume encode or decode operations from where it left off.
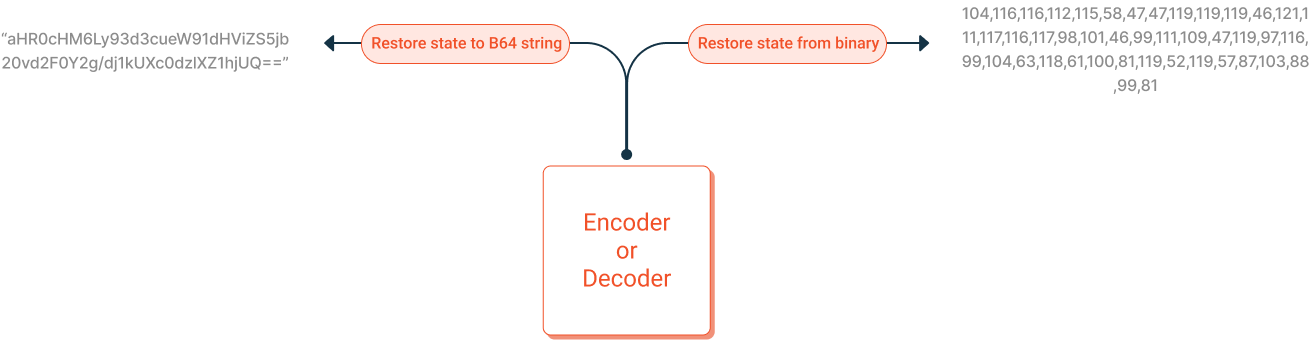
Encoder/Decoder Lifecycle
Managing the MTE state is a key part of a successful implementation. In a server application connecting to many clients, it is common only to want to initialize an Encoder or Decoder when it is being used.
For example, after an encode operation, you should save the Encoder's state and destroy the Encoder. This will preserve system resources (memory, CPU, etc.). When another encode operation needs to occur, you can create a new Encoder with default settings and restore its state using the previously saved state.
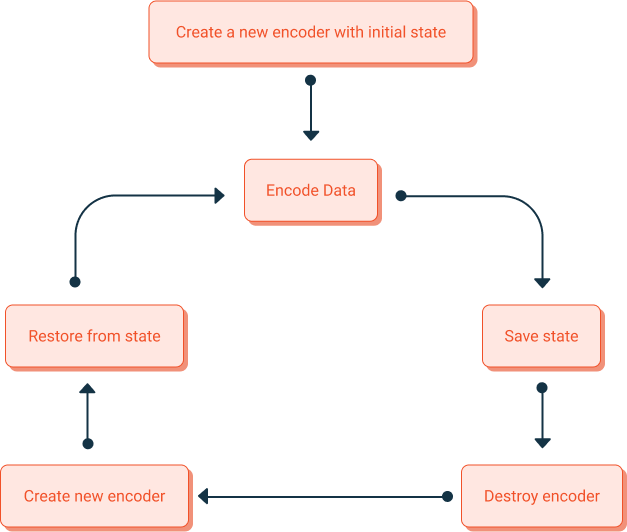
The above diagram of an MTE lifecycle is important for a server application. However, a client application rarely has more than a few Encoders/Decoders. Therefore in a client application, the recommended approach is to keep the Encoders/Decoders instantiated at all times.
103 - MTE, Fixed-Length MTE, and MKE
The MTE cryptographic library exports three types of Encoders and two types of Decoders, each with a specific use case. They are:
- MicroToken Exchange (MTE)
- Fixed-length MTE (FLEN)
- Managed Key Encryption (MKE)
MicroToken Exchange (MTE)
MTE Encoders and Decoders implement a proprietary, patented, and FIPs 140-3 certified encoding technology that replaces data with randomly generated, single-use values. MTE encoding is the most secure way to protect sensitive data before transmission.
MTE replaces each individual byte of original data with multiple bytes of randomly generated data. The number of replacement bytes is configurable, but recommended values are 8 or 16 bytes. For example, a payload of 1kb may be MTE encoded to a 16kb payload. This makes MTE highly secure for small values but not ideal for large payloads.

The diagram above shows how each byte of initial data is replaced with multiple bytes of randomly generated data. Larger replacement values create much larger MTE-encoded values.
Fixed-Length MTE (FLEN)
A normal MTE Encoder can secure data of any size, making it a very convenient solution. However, one vulnerability of MTE is that the size of the encoded data is directly proportional to the size of the original data. Malicious parties may observe packet sizes and attempt to infer information from them. To mitigate this, Fixed-Length (FLEN) MTE was created.
A Fixed-Length (FLEN) MTE Encoder includes a configuration option that allows the developer to set a fixed length of every encoded payload, regardless of the original data size. For example, if you set Fixed-Length to 64 bytes, MTE will pad out OR truncate your original data to exactly 64 bytes, then encode that data.
Fixed-Length MTE is the most secure way to use MTE; however, it is possible to try to encode a value larger than the fixed-length limit, in which case the original data will be truncated down to the fixed-length size, resulting in missing data.

Regardless of the size of the original data, the encoded value is always the same length. Encoded values that exceed the fixed-length limit will be truncated.
Managed Key Encryption (MKE)
Managed Key Encryption (MKE) is a module provided through the MTE library that utilizes industry-standard encryption algorithms to encrypt and decrypt data. MKE is special because it uses MTE technology to create new, single-use, randomly generated keys for each encryption and decryption event.
One of the benefits of MKE is that encrypted payloads are much closer in size to the size of the original value. This makes MKE the recommended approach for securing large payloads, especially file transfers.
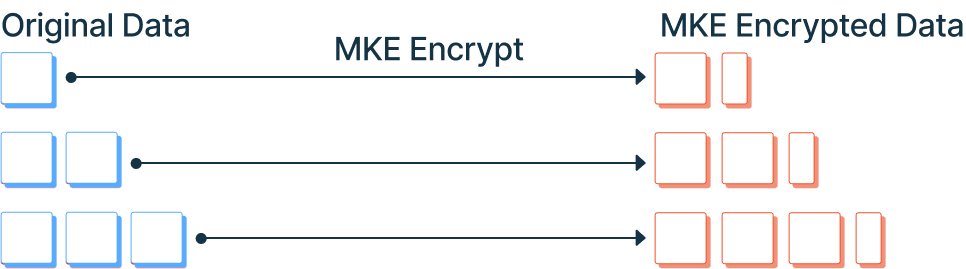
One of the disadvantages of MKE is that your data is only protected by industry-standard encryption algorithms, not by MTE itself. Rather, MTE technology creates a new encryption key for each new encryption event.